VirtualNet (over RMI)
Local example
- Declaring the interface
- Implementing the interface
- How to use virtual net and the server
- Executing the application
- Download the example
Declaring the interface
The local version of Virtual Net requires every server to implement an interface where it declares its services. This interface has to extend the vnet.local.VirtualRemote interface. There is another requirement: each of the methods of the interface has to throw the exception vnet.local.VirtualNetException
It is also possible that the server implements more than one interface; in this case, all these interfaces have to extend the vnet.local.VirtualRemote interface.
For this example, the server is going to give only one service: it returns a phone number, those used for its initialization.
The code for this interface is:
import vnet.*; import vnet.local.*; public interface PhoneNumbersIntf extends VirtualRemote { public String getNumber() throws VirtualNetException; }
Implementing the interface
Here is the code for a server that implements the previous interface:import vnet.*; import vnet.local.*; public class PhoneNumbersServer implements PhoneNumbersIntf { public PhoneNumbersServer(String number) { Number=number; } public String getNumber() throws vnet.VirtualNetException { return Number; } String Number; }
How to use virtual net and the server
The following is a very small apllication using the previous server. It creates some windows to follow the net state, and will finish with a communication exception.
import java.awt.Dimension; import vnet.*; import vnet.display.*; import vnet.local.*; public class PhoneProof { public static void main(String args[]) { try { System.out.println("Initializing"); VirtualNet net=new VirtualNet(); new BuildNetLayout("+a+e+i+m+o+a1e+e2i+e3m+i4m+m5o",net); new GraphNetFrame(new GraphNet(net,new GraphGeometryCircle()), "Local test", new Dimension(200,200)); new ConsoleNetFrame(net,"Local Test",new Dimension(300,300)); new ListBindsFrame(new ListBindsNet(net),"Binded servers", new Dimension(200,300)); System.out.println("Creating servers"); PhoneNumbersIntf pLmp=new PhoneNumbersServer("763.27.17"); PhoneNumbersIntf pSLmp=new PhoneNumbersServer("859.82.94"); System.out.println("Creating nodes"); NodeId a=new NodeId("a"); NodeId d=new NodeId("m"); NodeId e=new NodeId("o"); System.out.println("Binding servers"); net.bind(d,"LMP phone",pLmp); net.bind(e,"LMP phone",pSLmp); PhoneNumbersIntf found; String lookFor; lookFor=new String("LMP phone12"); System.out.println("Looking for "+lookFor); found=(PhoneNumbersIntf)net.lookup(a,lookFor); if (found==null) System.out.println("Not found"); lookFor=new String("LMP phone"); System.out.println("Looking for "+lookFor); found=(PhoneNumbersIntf)net.lookup(a,lookFor); if (found==null) System.out.println("Not found"); else System.out.println("telephone: "+found.getNumber()); System.out.println("Looking for "+lookFor+" in node specific"); found=(PhoneNumbersIntf)net.lookup(a,lookFor,e); if (found==null) System.out.println("Not found"); else System.out.println("telephone: "+found.getNumber()); System.out.println("Removing the link 5"); new BuildNetLayout("-5",net); System.out.println("Looking for "+lookFor+" in node specific"); found=(PhoneNumbersIntf)net.lookup(a,lookFor,e); if (found==null) System.out.println("Not found"); } catch(Exception e) { e.printStackTrace(); } } }
Executing the application
Before executing the application, the stub must be compiled and generated
javac PhoneNumbersIntf.java javac PhoneNumbersServer.java javac PhoneProof.java java vnet.local.vnc PhoneNumbersServer javac PhoneNumbersServer_VNet.java
It you get any compiler error, probably the classpath has not been properly set. In this case, go to the page on Availability to know how to set it. Now, to execute the application:
java PhoneProof
The console output for this small program will be:
Initializing Creating servers Creating nodes Binding servers Looking for LMP phone12 Not found Looking for LMP phone telephone: 763.27.17 Looking for LMP phone in node specific telephone: 859.82.94 Removing the link 5 Looking for LMP phone in node specific vnet.CommException: Communication failure at vnet.NetNode.checkWay(NetNode.java:277) at vnet.NetNode.lookup(NetNode.java:234) at vnet.local.VirtualNet.lookup(VirtualNet.java:131) at PhoneProof.main(PhoneProof.java:60)
It also generates three windows. Here there are some snapshots (they don't correspond exactly to this example):
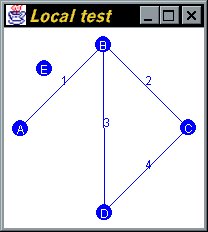
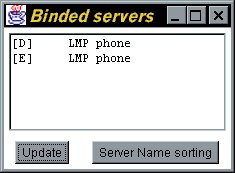
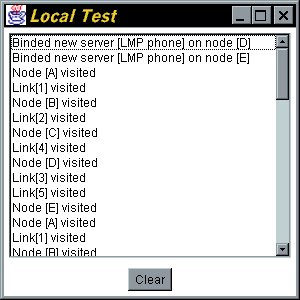
Download the example
vnetexloc.zip (5 KB)
Once unzipped this file -and having correctly set the classpath-, to execute the example one simply needs to:
java PhoneProof