VirtualNet (over RMI)
Overview
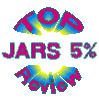
- What is Virtual Net
- Is it useful?
- Its philosophy: how to work with Virtual Net
- Client/Server application with Virtual Net
- Transparency with RMI
- An small example with RMI
- The Virtual Net Compiler
- Graphics
What is Virtual Net
Virtual Net is an application that allows the user to make a simulation of a network topology, and to run and test Client/Server programs on this net.
Is it useful?
Everybody can develop an RMI or CORBA Client/Server application and test it just on one machine, with the only difference of the access times; but, in this case, you can not just simulate network problems. This has been my main reason to develop this application, to be able to simulate network partitions.
How to work with Virtual Net
In Virtual Net, the net is defined by the nodes (the machines) and the links (its communication channels) between them.
On a real net, the servers and the client are attached to the machine where its process is being executed; is that hosting relation which is needed to simulate under Virtual Net. Therefore, any server or client, before giving or requesting any service, first has to get attached to any of the nodes. From that moment, the communications between the server and its clients are done throught the virtual net, using its defined nodes and links.
Client/Server applications with Virtual Net
On a client/server application, the server first has to be known; to do that, it has to register itself, and depending on the middleware, it can do it in very different ways. When it becomes registered, with a public name, any client could link to that server and therefore it could start making service requests.
To do this, there are three basic operations on Virtual Net:
- -Binding: the server is binded with an unique name to a host
- -Unbinding: the server is unbinded from the host
- -Lookup: the client looks for a server with an specified name
By now, vnet only allows to develop local C/S applications, and, much more interesting, RMI C/S applications.
VirtualNet2 is a port of this application to cover the same functionality under CORBA. In fact, VirtualNet2 addresses the problem under a different perspective and the result is much more effective.
Transparency with RMI
There is an additional feature that makes very interesting the use of this virtual net with RMI: its transparency, the changes to make to your C/S application is truly very small. You only have to think that any client or server first has to say in with node it is hosted. From that moment, no extra changes are needed.
Even the exceptions that can be thrown by Virtual Net have being mapped to the analogue RMI exceptions.
An small example with RMI
This is an small RMI program.
The interface:
public interface PhoneNumbersIntf extends java.rmi.Remote { public String getNumber() throws java.rmi.RemoteException; }
The implementation:
public class PhoneNumbersServer implements PhoneNumbersIntf { public PhoneNumbersServer(String number) { Number=number; } public String getNumber() throws java.rmi.RemoteException { return Number; } String Number; }
To use this server with Virtual Net, no changes are needed
In RMI, the implementation has to be compiled with rmic, which generates the stub and skeleton for the server. In VNet, it is still needed to execute rmic; but it is needed also an stub for the vnet, that is generated automatically by the virtual net compiler.
The only difference between an RMI and an RMI vnet application, is that, in the latter, the servers and clients have to be attached first (using the Naming service) to a node in the net (that is, they reside on a machine). From that moment, they will use the attached Host -that has the same interface as the Naming service- to bind, unbind or lookup a server.
RMI:
Naming.bind("LMP",new PhoneNumbersServer("763271")); PhoneNumbersIntf p=(PhoneNumbersIntf)Naming.lookup("LMP");
RMI Vnet:
Host host=(Host) Naming.lookup("/TestA/HostY"); host.bind("LMP",new PhoneNumbersServer("763271")); PhoneNumbersIntf p=(PhoneNumbersIntf)host.lookup("LMP");
And that's all!!
The Virtual Net Compiler
To get the stub, I have developped a very simple compiler that makes this task automatic.
There is a compiler for the local version, that needs as the only argument the class of the server, and that generates a java file that has to be compiled. This file has the same name as the server, with the suffix _VNet.
In the remote version, a stub is not needed for the server, but for its RMI stub. The compiler still needs to have the server itself, but it generates the stub directly for the RMI stub; in this case, the suffix is _Stub_VNet
Graphics
To follow the net state, it is possible to open the following windows:
- A console that shows all the events in the net.
- A list of binded servers.
- A graph representation. By now, there are two representations, one called net, and the another one, in circle. This representation even shows the messages passing, changing the colour of the affected nodes.
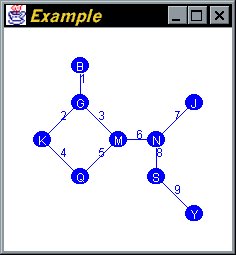