VirtualNet (over RMI)
RMI example
- Creating the server
- Generating servers
- Generating clients
- Executing the applications
- Download the example
Creating the server
The remote version of Virtual Net just need a RMI server; there are no special requirements of Virtual Net on the server.
For this example, the server is going to give only one service: it returns a phone number, those used for its initialization.
The code for this interface is:
public interface PhoneNumbersIntf extends java.rmi.Remote { public String getNumber() throws java.rmi.RemoteException; }
The code for the server is:
public class PhoneNumbersServer extends java.rmi.server.UnicastRemoteObject implements PhoneNumbersIntf { public PhoneNumbersServer(String number) throws java.rmi.RemoteException { Number=number; } public String getNumber() throws java.rmi.RemoteException { return Number; } String Number; }
Generating servers
The following is the code that generates several servers:
import java.rmi.Naming; import vnet.remote.Host; public class InsertServers { public static void main(String args[]) { try { System.out.println("This test expects to have a virtualNet called " +"TestA, with 4 nodes C,M,U,Y, with links between C-M, M-U, V-Y"); System.out.println(); System.out.println("Getting the hosts..."); Host C=(Host) Naming.lookup("/TestA/HostC"); Host M=(Host) Naming.lookup("/TestA/HostM"); Host U=(Host) Naming.lookup("/TestA/HostU"); Host Y=(Host) Naming.lookup("/TestA/HostY"); System.out.println("Binding the servers"); System.out.println("In M there is a server called LMP"); M.bind("LMP",new PhoneNumbersServer("763271")); System.out.println("In Y there is the same server ,but with a "+ "different number"); Y.bind("LMP",new PhoneNumbersServer("859829")); System.out.println("In C there is a server, hosted in U, called "+ "BELLMP (/TestA/HostC/BELLMP"); U.bind("/TestA/HostC/BELLMP", new PhoneNumbersServer("213207")); System.out.println(); System.out.println(); System.out.println("Ready"); } catch(Exception ex) { System.out.println(ex); } } }
Generating clients
The following is a very small apllication that acts as a client for the previous generated clients.
import java.rmi.Naming; import vnet.remote.Host; public class CheckServers { public static void main(String args[]) { System.out.println("This test expects to have a virtualNet called TestA, with " + " 4 nodes C,M,U,Y, with links between C-M, M-U, V-Y"); if (args.length!=1) System.out.println("This test need an argument: The letter of the host from "+ "where the tests are done"); else try { System.out.println("Getting the host"); Host host=(Host) Naming.lookup("/TestA/Host"+args[0]); PhoneNumbersIntf phone=null; System.out.println("getting LMP"); phone=(PhoneNumbersIntf)host.lookup("LMP"); System.out.println("phone: "+phone.getNumber()); System.out.println("getting /TestA/HostY/LMP"); phone=(PhoneNumbersIntf)host.lookup("/TestA/HostY/LMP"); System.out.println("phone: "+phone.getNumber()); System.out.println("getting /TestA/HostM/LMP"); phone=(PhoneNumbersIntf)host.lookup("/TestA/HostM/LMP"); System.out.println("phone: "+phone.getNumber()); System.out.println("getting BELLMP"); phone=(PhoneNumbersIntf)host.lookup("BELLMP"); System.out.println("phone: "+phone.getNumber()); System.out.println("getting /TestA/HostC/BELLMP"); phone=(PhoneNumbersIntf)host.lookup("/TestA/HostC/BELLMP"); System.out.println("phone: "+phone.getNumber()); System.out.println("getting /TestA/HostU/BELLMP"); phone=(PhoneNumbersIntf)host.lookup("/TestA/HostU/BELLMP"); System.out.println("phone: "+phone.getNumber()); } catch(Exception ex) { System.out.println(ex); } } }
Executing the application
In the RMI remote case, there are four parts:
- The RMI system
- The Virtual Net application
- One or more servers
- One or more clients
First one has to compile the java files:
javac PhoneNumbersIntf.java javac PhoneNumbersServer.java javac InsertServers.java javac CheckServers.java
It you get any compiler error, probably the classpath has not been properly set. In this case, go to the page on Availability to know how to set it. Then, we start the RMI registry:
start rmiregistry (rmiregistry & in Unix)
Now, we need to have the Virtual Net application, and to initialize a net called TestA that will be used by the servers and clients.
There are several ways to execute this application. One of them is generating another program that creates an object vnet.remote.VirtualNet.
Another way is to use one of the two programs hosted in vnet.remote.prg. We will use the graphic version:
javaw vnet.remote.prg.GraphMain -init=+C+M+U+Y+MU1+MC2+MY3 -start TestA
The graph of this net is:
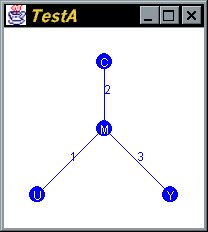
Before executing the server or client applications, you have to generate the stub for the server, and its stub and skeleton:
rmic PhoneNumbersServer java vnet.remote.vnc PhoneNumbersServer javac PhoneNumbersServer_Stub_VNet.java rmic PhoneNumbersServer_Stub_VNet
Now, it is already possible to install the servers:
java InsertServers
The output of this program will be:
This test expects to have a virtualNet called TestA, with 4 nodes C,M,U,Y, with links between C-M, M-U, V-Y Getting the hosts... Binding the servers In M there is a server called In Y there is the same server ,but with a different number In C there is a server, hosted in U, called BELLMP (/TestA/HostC/BELLMP Ready
Finally, we test the client:
java CheckServers C
The ouput is:
This test expects to have a virtualNet called TestA, with 4 nodes C,M,U,Y, with links between C-M, M-U, V-Y Getting the host getting LMP phone: 763271 getting /TestA/HostY/LMP phone: 859829 getting /TestA/HostM/LMP phone: 763271 getting BELLMP phone: 213207 getting /TestA/HostC/BELLMP phone: 213207 getting /TestA/HostU/BELLMP java.rmi.NotBoundException: /TestA/HostU/BELLMP
Download the example
vnetexrmi.zip (10 KB)
Once unzipped this file -and having correctly set the classpath-, to execute the example is just needed to do:
start rmiregistry (rmiregistry & in Unix) javaw vnet.remote.prg.GraphMain -init=+C+M+U+Y+MU1+MC2+MY3 -start TestA start java InsertServers (java InsertServers & in Unix) java CheckServers C